Understanding Interfaces in Java Lesson 62 Java Programming Learning Programming
YOUR LINK HERE:
http://youtube.com/watch?v=Noq9U6_6nmU
Understanding Interfaces in Java • In this class, We discuss Understanding Interfaces in Java. • The reader should have prior knowledge of abstract classes and methods. Click Here. • Interface: • An interface is a fully abstract class. • The interface contains abstract methods and static constants. • Example: • interface A • { • int p=20; • void m1(); • } • Class B implements A • { • public void m1() • { • System.out.println(“m1 method implemented in B”); • } • } • class test • { • public static void main(String args[]) • { • B ob = new B(); • ob.m1(); • } • } • The Java compiler will convert void m1(); to public abstract void m1(); because A is an interface. • Similarly, int p = 20 is converted to public static final int p = 20; • The compiler adds the extra code. • To inherit the interface, we use the keyword “implements”. • Suppose a class implements an interface. Then all the methods should be implemented. • Interfaces can have static methods. • Example: • interface A • { • int p=20; • public static void staticmethod() • { • System.out.println(“static method”); • } • void m1(); • } • Class B implements A • { • public void m1() • { • A.staticmethod(); • System.out.println(“m1 method implemented in B”); • } • } • class test • { • public static void main(String args[]) • { • B ob = new B(); • ob.m1(); • } • } • Inheritance for interfaces • interface A • { • int p=20; • void m1(); • } • interface B extends A • { • void m2(); • } • Class C implements B • { • public void m1() • { • System.out.println(“m1 method implemented in B”); • } • public void m2() • { • System.out.println(“m2 method”); • } • } • class test • { • public static void main(String args[]) • { • C ob = new C(); • ob.m1(); • } • } • Yes, inheritance is applied for interfaces. • Link for playlists: • / @learningmonkey • • Link for our website: https://learningmonkey.in • Follow us on Facebook @ / learningmonkey • Follow us on Instagram @ / learningmonkey1 • Follow us on Twitter @ / _learningmonkey • Mail us @ [email protected]
#############################
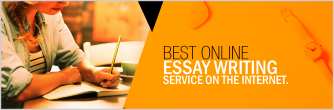