Processing thousands amp millions of files media deduplication python coding programming tips
>> YOUR LINK HERE: ___ http://youtube.com/watch?v=RmB-u4HtHW4
1. Understanding the Challenge • File Types: Media files can be in various formats (JPEG, PNG, MP4, MP3, etc.), which adds complexity. • File Sizes: Media files are usually large, making processing time and memory management critical. • Duplication Types: Duplicates can be exact (same file) or near-duplicates (similar files but with minor differences like different resolutions). • 2. Tools and Libraries • Several Python libraries can help with media file processing and deduplication: • PIL/Pillow: For image processing. • OpenCV: For more advanced image processing. • FFmpeg: For video and audio processing. • ImageHash: For generating perceptual hashes of images. • pHash (Perceptual Hashing): For comparing images/videos based on visual content. • 3. General Workflow for Media Deduplication • 1. Preprocessing and Loading Files • File Collection: Gather all media files from directories, subdirectories, or storage solutions like cloud buckets. • Batch Processing: If the dataset is large, process the files in batches to avoid memory overload. • python • Copy code • import os • def get_files(directory, extensions): • media_files = [] • for root, dirs, files in os.walk(directory): • for file in files: • if file.endswith(extensions): • media_files.append(os.path.join(root, file)) • return media_files • media_files = get_files('/path/to/media/files', ('.jpg', '.png', '.mp4')) • 2. File Hashing (Exact Deduplication) • MD5/SHA-256 Hashing: Generate a hash of each file. Identical files will produce the same hash. • python • Copy code • import hashlib • def file_hash(file_path): • hash_md5 = hashlib.md5() • with open(file_path, rb ) as f: • for chunk in iter(lambda: f.read(4096), b ): • hash_md5.update(chunk) • return hash_md5.hexdigest() • unique_files = {} • for file in media_files: • hash_value = file_hash(file) • if hash_value not in unique_files: • unique_files[hash_value] = file • else: • print(f Duplicate found: {file} and {unique_files[hash_value]} ) • 3. Perceptual Hashing (Near-Duplicate Deduplication) • ImageHash for Images: Generate perceptual hashes that account for visual similarity rather than exact file matches. • python • Copy code • from PIL import Image • import imagehash • def get_image_hash(file_path): • image = Image.open(file_path) • return imagehash.average_hash(image) • unique_images = {} • for file in media_files: • hash_value = get_image_hash(file) • if hash_value not in unique_images: • unique_images[hash_value] = file • else: • print(f Near-duplicate found: {file} and {unique_images[hash_value]} ) • pHash for Videos: Generate perceptual hashes for video frames to detect near-duplicate videos. • python • Copy code • import cv2 • import imagehash • from PIL import Image • def video_frame_hash(video_path, frame_number=30): • vidcap = cv2.VideoCapture(video_path) • vidcap.set(cv2.CAP_PROP_POS_FRAMES, frame_number) • success, image = vidcap.read() • if success: • pil_image = Image.fromarray(cv2.cvtColor(image, cv2.COLOR_BGR2RGB)) • return imagehash.average_hash(pil_image) • return None • unique_videos = {} • for file in media_files: • hash_value = video_frame_hash(file) • if hash_value and hash_value not in unique_videos: • unique_videos[hash_value] = file • else: • print(f Near-duplicate video found: {file} and {unique_videos[hash_value]} ) • 4. Clustering Similar Files • Clustering Algorithms: For large datasets, use clustering algorithms (e.g., K-means) to group similar files based on their hashes or other features like color histograms, SIFT features, or audio spectrograms. • Dimensionality Reduction: Use PCA or t-SNE to reduce feature space dimensions before clustering. • python • Copy code • from sklearn.cluster import KMeans • import numpy as np • Example with image hashes converted to a numerical format • image_hashes = np.array([hash_value.hash.flatten() for hash_value in unique_images.keys()]) • kmeans = KMeans(n_clusters=10).fit(image_hashes) • clusters = {i: [] for i in range(10)} • for idx, label in enumerate(kmeans.labels_): • clusters[label].append(media_files[idx]) • 5. Parallel Processing • Multiprocessing: Use Python’s multiprocessing module to parallelize the hashing and comparison process, especially when dealing with millions of files. • python • Copy code • from multiprocessing import Pool • def process_file(file): • return file, get_image_hash(file) • with Pool() as pool: • results = pool.map(process_file, media_files) • unique_files = {} • for file, hash_value in results: • if hash_value not in unique_files: • unique_files[hash_value] = file • else: • print(f Duplicate found: {file} and {unique_files[hash_value]} ) • 6. Handling Large-Scale Data • Distributed Systems: For massive datasets, consider using distributed processing frameworks like Apache Spark with PySpark to handle the deduplication at scale. • Databases: Store hashes and metadata in a database like PostgreSQL, MongoDB","styleRuns":[{"startIndex":0,"length":3779,"styleRunExtensions":{"styleRunColorMapExtension":{"colorMap":[{"key":"USER_INTERFACE_THEME_DARK","value":4294967295},{"key":"USER_INTERFACE_THEME_LIGHT","value":4279440147}]}},"fontFamilyName":"Roboto"},{"startIndex":3779,"length":57,"fontSize":0,"styleRunExtensions":{"styleRunColorMapExtension":{"colorMap":[{"key":"USER_INTERFACE_THEME_DARK","value":4294967295},{"key":"USER_INTERFACE_THEME_LIGHT","value":4279440147}]}},"fontFamilyName":"Roboto"},{"startIndex":3836,"length":1110,"styleRunExtensions":{"styleRunColorMapExtension":{"colorMap":[{"key":"USER_INTERFACE_THEME_DARK","value":4294967295},{"key":"USER_INTERFACE_THEME_LIGHT","value":4279440147}]}},"fontFamilyName":"Roboto"}]},"headerRuns":[{"startIndex":0,"length":3779,"headerMapping":"ATTRIBUTED_STRING_HEADER_MAPPING_UNSPECIFIED"},{"startIndex":3779,"length":57,"headerMapping":"ATTRIBUTED_STRING_HEADER_MAPPING_HEADING_1"},{"startIndex":3836,"length":1110,"headerMapping":"ATTRIBUTED_STRING_HEADER_MAPPING_UNSPECIFIED"}]}},{"itemSectionRenderer":{"contents":[{"continuationItemRenderer":{"trigger":"CONTINUATION_TRIGGER_ON_ITEM_SHOWN","continuationEndpoint":{"clickTrackingParams":"CLIBELsvGAIiEwjn7f7J3MiLAxXVWHoFHQxgJd8=","commandMetadata":{"webCommandMetadata":{"sendPost":true,"apiUrl":"/youtubei/v1/next"}},"continuationCommand":{"token":"Eg0SC1JtQi11NEh0SFc0GAYyJSIRIgtSbUItdTRIdEhXNDAAeAJCEGNvbW1lbnRzLXNlY3Rpb24%3D","request":"CONTINUATION_REQUEST_TYPE_WATCH_NEXT"}}}}],"trackingParams":"CLIBELsvGAIiEwjn7f7J3MiLAxXVWHoFHQxgJd8=","sectionIdentifier":"comment-item-section","targetId":"comments-section"}}],"trackingParams":"CLEBELovIhMI5-3-ydzIiwMV1Vh6BR0MYCXf"}},"secondaryResults":{"secondaryResults":{"results":[{"lockupViewModel":{"contentImage":{"collectionThumbnailViewModel":{"primaryThumbnail":{"thumbnailViewModel":{"image":{"sources":[{"url":"https://i.ytimg.com/vi/jbT3baUkdfc/hqdefault.jpg?sqp=-oaymwEwCKgBEF5IWvKriqkDIwgBFQAAiEIYAfABAfgBtgiAAoAPigIMCAAQARhBIGAoZTAP rs=AOn4CLCoTVyapx2qQRA3mQMDVRDsMMYx2A","width":168,"height":94},{"url":"https://i.ytimg.com/vi/jbT3baUkdfc/hqdefault.jpg?sqp=-oaymwExCNACELwBSFryq4qpAyMIARUAAIhCGAHwAQH4AbYIgAKAD4oCDAgAEAEYQSBgKGUwDw== rs=AOn4CLDLDNi-7zy9OHABsOAcHQzJCza2zA","width":336,"height":188}]},"overlays":[{"thumbnailOverlayBadgeViewModel":{"thumbnailBadges":[{"thumbnailBadgeViewModel":{"icon":{"sources":[{"clientResource":{"imageName":"PLAYLISTS"}}]},"text":"65 videos","badgeStyle":"THUMBNAIL_OVERLAY_BADGE_STYLE_DEFAULT","backgroundColor":{"lightTheme":1582118,"darkTheme":1582118}}}],"position":"THUMBNAIL_OVERLAY_BADGE_POSITION_BOTTOM_END"}},{"thumbnailHoverOverlayViewModel":{"icon":{"sources":[{"clientResource":{"imageName":"PLAY_ALL"}}]},"text":{"content":"Play all","styleRuns":[{"startIndex":0,"length":8}]},"style":"THUMBNAIL_HOVER_OVERLAY_STYLE_COVER"}}],"backgroundColor":{"lightTheme":2636863,"darkTheme":2636863}}},"stackColor":{"lightTheme":7049625,"darkTheme":7374732}}},"metadata":{"lockupMetadataViewModel":{"title":{"content":"Python Tutorials and Tips"},"metadata":{"contentMetadataViewModel":{"metadataRows":[{"metadataParts":[{"text":{"content":"Tech Keys X
#############################
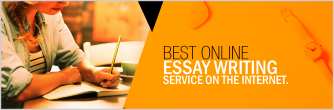